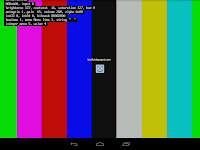
This example is the same as AugmentedRealityExample using 2 files in org.anddev.andengine.extension.augmentedreality package
Create new class named AugmentedRealityHorizonExample in org.anddev.andengine.examples package. Here is the code of this class:
package org.anddev.andengine.examples;import org.anddev.andengine.engine.Engine;import org.anddev.andengine.engine.camera.Camera;import org.anddev.andengine.engine.options.EngineOptions;import org.anddev.andengine.engine.options.EngineOptions.ScreenOrientation;import org.anddev.andengine.engine.options.resolutionpolicy.RatioResolutionPolicy;import org.anddev.andengine.entity.scene.Scene;import org.anddev.andengine.entity.scene.background.ColorBackground;
Open the AndroidManifest.xml and append the activity element below the previous activity element like this:import org.anddev.andengine.entity.sprite.Sprite;import org.anddev.andengine.entity.util.FPSLogger;import org.anddev.andengine.extension.augmentedreality.BaseAugmentedRealityGameActivity;import org.anddev.andengine.opengl.texture.TextureOptions;import org.anddev.andengine.opengl.texture.atlas.bitmap.BitmapTextureAtlas;import org.anddev.andengine.opengl.texture.atlas.bitmap.BitmapTextureAtlasTextureRegionFactory;import org.anddev.andengine.opengl.texture.region.TextureRegion;import org.anddev.andengine.sensor.orientation.IOrientationListener;import org.anddev.andengine.sensor.orientation.OrientationData;import org.anddev.andengine.util.Debug;import android.widget.Toast;/*** (c) 2010 Nicolas Gramlich* (c) 2011 Zynga** @author Nicolas Gramlich* @since 11:54:51 - 03.04.2010*/public class AugmentedRealityHorizonExample extends BaseAugmentedRealityGameActivity implements IOrientationListener {// ===========================================================// Constants// ===========================================================private static final int CAMERA_WIDTH = 720;private static final int CAMERA_HEIGHT = 480;// ===========================================================// Fields// ===========================================================private Camera mCamera;private BitmapTextureAtlas mBitmapTextureAtlas;private TextureRegion mFaceTextureRegion;private Sprite mFace;// ===========================================================// Constructors// ===========================================================// ===========================================================// Getter & Setter// ===========================================================// ===========================================================// Methods for/from SuperClass/Interfaces// ===========================================================@Overridepublic Engine onLoadEngine() {Toast.makeText(this, "If you don't see a sprite moving over the screen, try starting this while already being in Landscape orientation!!", Toast.LENGTH_LONG).show();this.mCamera = new Camera(0, 0, CAMERA_WIDTH, CAMERA_HEIGHT);return new Engine(new EngineOptions(true, ScreenOrientation.LANDSCAPE, new RatioResolutionPolicy(CAMERA_WIDTH, CAMERA_HEIGHT), this.mCamera));}@Overridepublic void onLoadResources() {this.mBitmapTextureAtlas = new BitmapTextureAtlas(32, 32, TextureOptions.BILINEAR_PREMULTIPLYALPHA);BitmapTextureAtlasTextureRegionFactory.setAssetBasePath("gfx/");this.mFaceTextureRegion = BitmapTextureAtlasTextureRegionFactory.createFromAsset(this.mBitmapTextureAtlas, this, "face_box.png", 0, 0);this.mEngine.getTextureManager().loadTexture(this.mBitmapTextureAtlas);}@Overridepublic Scene onLoadScene() {this.mEngine.registerUpdateHandler(new FPSLogger());final Scene scene = new Scene();// scene.setBackgroundEnabled(false);scene.setBackground(new ColorBackground(0.0f, 0.0f, 0.0f, 0.0f));final int centerX = (CAMERA_WIDTH - this.mFaceTextureRegion.getWidth()) / 2;final int centerY = (CAMERA_HEIGHT - this.mFaceTextureRegion.getHeight()) / 2;this.mFace = new Sprite(centerX, centerY, this.mFaceTextureRegion);scene.attachChild(this.mFace);return scene;}@Overridepublic void onLoadComplete() {this.enableOrientationSensor(this);}@Overridepublic void onOrientationChanged(final OrientationData pOrientationData) {final float roll = pOrientationData.getRoll();Debug.d("Roll: " + pOrientationData.getRoll());this.mFace.setPosition(CAMERA_WIDTH / 2, CAMERA_HEIGHT / 2 + (roll - 40) * 5);}// ===========================================================// Methods// ===========================================================// ===========================================================// Inner and Anonymous Classes// ===========================================================}
<activityandroid:name=".AugmentedRealityExample"android:label="@string/app_name" ><intent-filter><action android:name="org.anddev.andengine.examples.AUGMENTEDREALITYEXAMPLE" /><category android:name="android.intent.category.DEFAULT" /></intent-filter></activity><activityandroid:name=".AugmentedRealityHorizonExample"android:label="@string/app_name" ><intent-filter><action android:name="org.anddev.andengine.examples.AUGMENTEDREALITYHORIZONEXAMPLE" /><category android:name="android.intent.category.DEFAULT" /></intent-filter></activity>
Run the project (in debug mode) to re-generate apk file in the bin folderOpen and Edit the batch command file named r.bat in the bin folder with some commands like this:
adb install -r AndEngineDemo.apk
adb shell am start -n org.anddev.andengine.examples/.AugmentedRealityHorizonExample
Run android emulator with virtualbox
Then run the batch file r.bat
The result's like this with animated pictures
Then run the batch file r.bat
The result's like this with animated pictures
[ttaiit.blogspot.com] - please write clearly the source on copying this tutorial :)
0 comments:
Post a Comment